Basic fundamental about react-router with simple react-router example. In this tutorial we learn how you will create a custom URL in react application. Basically in React.js react-router is a routing library which responsible for routing react component and synchronize with location URL.
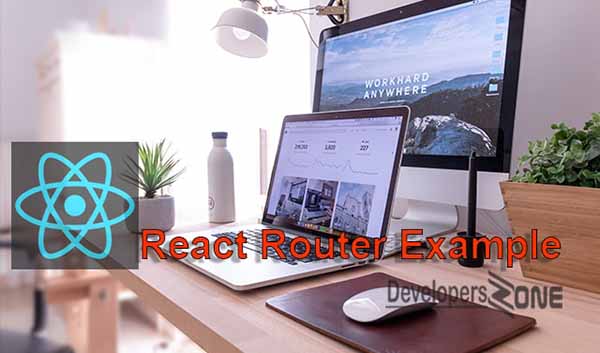
In this tutorial we will discuss how to navigate your web page via URL in react js. Also we create a navigation menu with react.js with some simple example.
Why React Router important for a web application?
In react application routing of component is very important. Because if you create multiple components in the application then it’s very difficult to navigate each component. And without routing you are not able to navigate the app component by URL. So react-router is important.
Now try to understand the react-router library with a simple react-router example. For example we will create some simple components like “Home, AboutUs, Service, and Products” and try to navigate via URL using the library.
Failed to compile
./src/App.js
Attempted import error: 'browserHistory' is not exported from 'react-router-dom'.
If you got the above error the make sure that you have install react-router v3 or higher if available. To Install react-router use the command below.
npm install react-router@3
You can also read
Best and Simple way to Install React
Click on above link to Install React
Best Example of React Component
Click to know how you will create react component.
React.js in Visual Studio Code
First of all create a directory under “src” in this example we create a “pages” directory under “src”. Then create javascript files like “Home, AboutUs, Service and Products”. Please see the sample code of the above file.
Sample code for the “App.js” file which basically contains the react-router library and sample routing code.
“App.js” file
“Home.js” file
“Services.js” file
“Products.js” file
Output of above react router example
- Home
- About Us
- Services
- Products
You can navigate by clicking on the link .