Today we will learn to view pdf in the flutter with flutter_pdfview. Using this plugin we can open a pdf from the URL. And we don’t need to download or store it in the local storage.
Plugin: Here we use the flutter_pdfview plugin. With this package, we can view pdf from URLs as well as from local files in our Flutter projects.
It has 120 pub points and 99% popularity in Flutter.
Table of Content
- Install the flutter_pdfview Plugin in Your Project
- Import the Pdf Viewer Package
- View pdf from URL in the Flutter
Step – 1: Install the flutter_pdfview Plugin in Your Project
Step -1.1: Add the dependency to the pubspec.yaml file
Go to the pub.dev. And type flutter_pdfview. Then you find the packages and copy the dependency. We should use the latest version and also null safety-type packages. Then paste it into pubspec.yaml file.
We also add HTTP Packages to our projects.
dependencies:
flutter:
sdk: flutter
cupertino_icons: ^1.0.2
http: ^0.13.5
Step -1.2: Run Flutter pub get command
After adding the packages, we go to the terminal, type the flutter pub get command, and hit the enter button.
flutter pub get
Step – 2: Import the Pdf Viewer Package
Now import the package to that page when we want to write the code for creating a pdf viewer button.
flutter_pdfview: ^1.2.1
Then run flutter pub get command again.
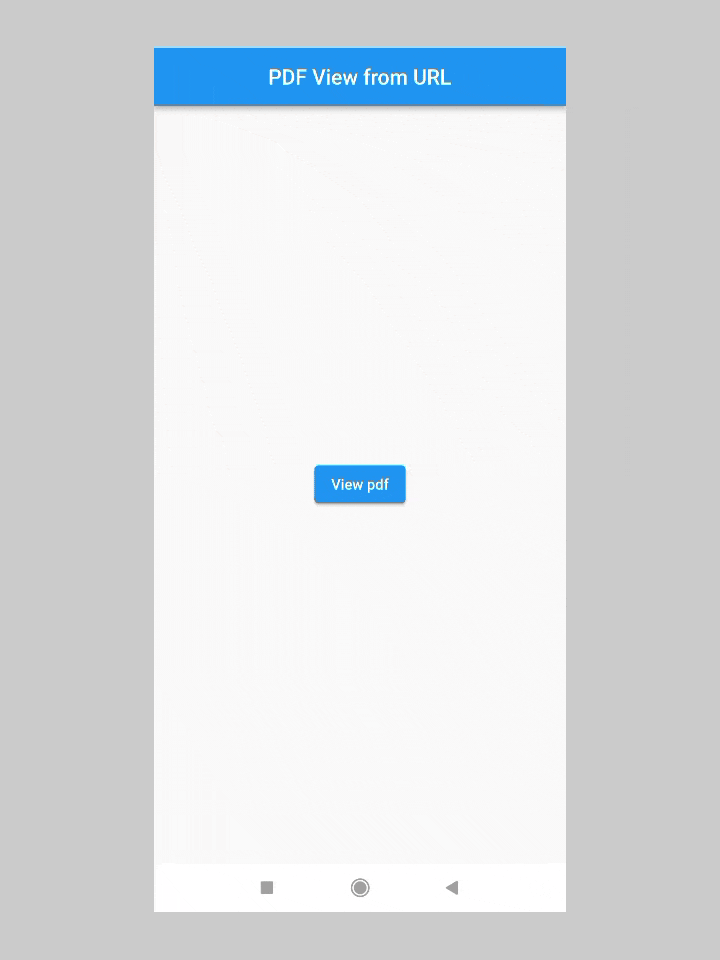
Step – 3: Codes to view pdf from URL in the Flutter
Step -3.1: Create a Button to view Pdf in the Flutter
In our main.dart page we create a Stateful widget. Then in the Stateful widget, we create a Scaffold widget. And in the Scaffold’s body, we create a center widget. and in the center widget, we make an Elevated button.
Step -3.2: Permissions to view pdf from URL
Here we need to do a setup for android and ios devices. Basically, we need to add some permissions.
For Androids: Open the android folder. Go to the AndroidManifest file. And add these permissions.
<uses-permission android:name="android.permission.WAKE_LOCK" />
<uses-permission android:name="android.permission.INTERNET"/>
For IOS: Go to the ios folder. And open the info.plist folder. Then add this key-value pair.
<key>CFBundleDevelopmentRegion</key>
Step -3.3: Write Codes for the pdf viewer
We create two pages—one, the main file, for executing the function to view pdf. And the second one, named pdfviewPage, is created for viewing pdf.
Main Page:
import 'package:flutter/material.dart';
import 'package:pdf_view_app/pdfPage.dart';
void main() {
runApp(const MyApp());
}
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return MaterialApp(
home: HomePage(),
debugShowCheckedModeBanner: false,
);
}
}
class HomePage extends StatefulWidget {
const HomePage({Key? key}) : super(key: key);
@override
State<HomePage> createState() => _HomePageState();
}
class _HomePageState extends State<HomePage> {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("PDF View from URL"),
centerTitle: true,
),
body: Center(
child: ElevatedButton(
onPressed: () {
Navigator.push(context,
MaterialPageRoute(builder: (context) => PdfViewerPage()));
},
child: Text("View pdf"),
),
),
);
}
}
PdfView Page:
import 'package:flutter/material.dart';
import 'package:flutter_pdfview/flutter_pdfview.dart';
import 'dart:io';
import 'package:http/http.dart' as http;
import 'package:path/path.dart';
import 'package:path_provider/path_provider.dart';
class PdfViewerPage extends StatefulWidget {
@override
_PdfViewerPageState createState() => _PdfViewerPageState();
}
class _PdfViewerPageState extends State<PdfViewerPage> {
late File Pfile;
bool isLoading = false;
Future<void> loadNetwork() async {
setState(() {
isLoading = true;
});
var url = 'http://www.pdf995.com/samples/pdf.pdf';
final response = await http.get(Uri.parse(url));
final bytes = response.bodyBytes;
final filename = basename(url);
final dir = await getApplicationDocumentsDirectory();
var file = File('${dir.path}/$filename');
await file.writeAsBytes(bytes, flush: true);
setState(() {
Pfile = file;
});
print(Pfile);
setState(() {
isLoading = false;
});
}
@override
void initState() {
loadNetwork();
super.initState();
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text(
"Flutter PDF Viewer",
style: TextStyle(fontWeight: FontWeight.bold),
),
),
body: isLoading
? Center(child: CircularProgressIndicator())
: Container(
child: Center(
child: PDFView(
filePath: Pfile.path,
),
),
),
);
}
}
I will right away grasp your rss as I can not to find your e-mail subscription link or e-newsletter service. Do you have any? Kindly let me recognise so that I could subscribe. Thanks.