Getting a front-end job? React JS is usually the showstopper of the current tech interviews. Whether you are a fresher or a veteran developer, the React interview can present some challenging questions to you. Don’t worry, though—this easy, entertaining, and practical guide has got your back.
INDEX
- Learn the Basics Before Diving into Hooks
- React interview Master like best friends
- React interview Questions Coding Practice
- Familiarize Yourself with Common React Interview Questions
- Learn a Little Redux (Even if You Hate It)
- Understand How React Interacts with APIs and Routing
- Don’t Just Code—Explain Your Thinking Clearly
- Bonus: Soft Skills Will Also Help You Nail the React Interview
- Final Words: Practice, Patience, and Positivity
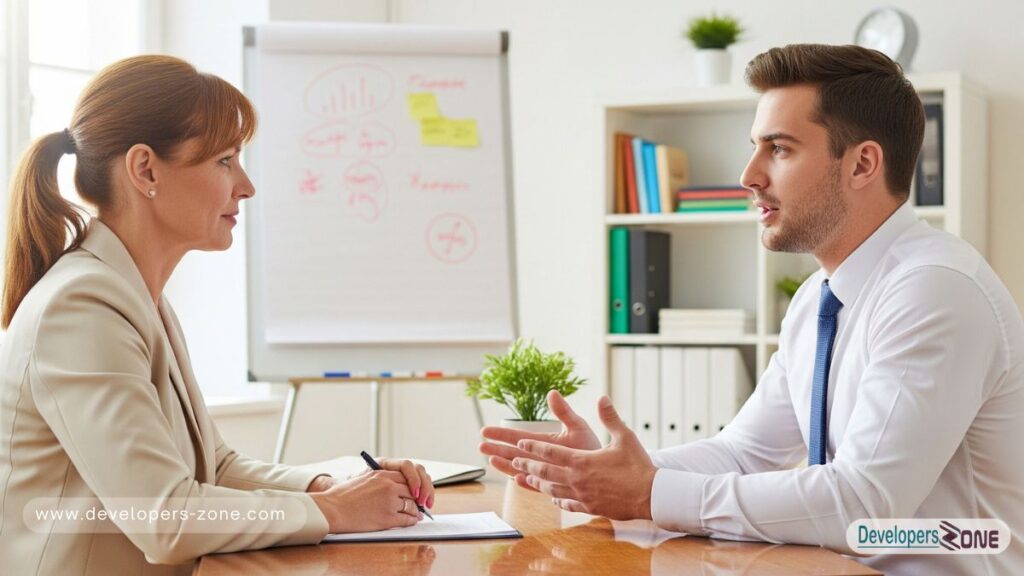
1. Learn the Basics Before Diving into Hooks
You don’t have to memorize all of the React documentation—but having the basics down helps a lot in any React interview round.
Begin with JSX, the core of all React components. Understand how it allows you to write HTML in JavaScript so easily.
Then learn about components—both class and functional. Despite the fact that functional components are the go-to, class components still arise in interviews.
React props may seem dull, but they are mighty. Consider them as the adhesives between components holding your UI in constant conversation with itself.
State is the journal of your component. It records whatever changes. Master how to update and handle it correctly.
And, learn the distinction between props and state. Interviewers adore asking this old-school deceptively tricky question—be prepared to flaunt.
Don’t ignore event handling. Clicks, input, mouse-over—React does things its own way with these browser events.
Conditional rendering is another favorite. Can you conditionally hide a button or display a message? You should be able to.
You should also get to know lists and keys. If you ever map over data, keys assist React in knowing what’s changed or added.
Lastly, controlled components and forms are always involved. Practice dealing with user inputs and staying in sync.
Master these, and you’re already halfway there to acing your React interview.
2. React interview Master like best friends
Hooks are the superheroes of React in modern times. Master them well—they’re a surefire requirement for every React interview nowadays.
Begin with the renowned useState
. It makes your components remember something such as a counter or a user’s name.
Then there’s the intro to useEffect
. It does it all—data fetching, side effects, and cleanup when necessary.
You should understand how dependencies are done in useEffect
. It is so easy to get it wrong. And yes, they will be asking for it.
Exercise writing a useEffect
that loads an API only once, and one that responds to a change in props or state.
And then there is useContext
. Eliminates prop drilling headache. Learn how to pass data between components the intelligent way.
Don’t memorize syntax—construct little examples. Interviewers prefer real-world insight, not textbook responses.
Make a simple app: a theme toggle based on context. You’ll impress your interviewer, and you’ll also learn quicker.
Custom hooks are another buzzworthy subject. They allow you to reuse logic in a clean and readable manner. Learn to construct one or two.
You might also see useRef
, useMemo
, and useCallback
. They’re higher-level, but try to learn the fundamental application of what each one does.
If you can demonstrate why and when to apply a specific hook, your confidence in the React interview room will be great.
3. React interview Questions Coding Practice
A good React interview is not all about theory. Coding rounds challenge how you think, not merely what you do.
Try challenges on sites like LeetCode, CodeSignal, or Frontend Mentor. Look for tasks that involve lists, forms, and data.
Start small. Build a to-do list app with add and delete buttons. That’s React in a nutshell—and a great test of skills.
Then challenge yourself. Build a weather app using an API. You’ll touch useEffect, loading states, and conditional rendering.
Build a movie search app with filters. React enjoys these types of puzzles—and so do interviewers.
If you’re preparing for a React interview, check out this Reddit thread for practical advice and insights.
Remember to time yourself. Interviews are brief, and timing is everything. Practice writing neat code fast and efficiently.
Utilize CodeSandbox or StackBlitz for immediate feedback. It makes you debug quicker and identify syntax errors with ease.
Solve one React puzzle each day for 15–30 minutes. It accumulates quickly and keeps your mind nimble.
Maintain a habit tracker for your progress. When you reflect, you will be surprised at how far you have progressed in such a short span of weeks.
4. Familiarize Yourself with Common React Interview Questions
Let’s address some questions that come up every time. If you practice these, you’ll go in prepared and smiling.
- What is the virtual DOM? Describe how React updates a virtual copy of the DOM first, then efficiently syncs it with the actual DOM.
- What’s the difference between functional and class components? Mention that functional components are cleaner and can use hooks, while class components use lifecycle methods.
- Explain the useEffect hook. Give a simple use case—like fetching data or setting up a timer. Don’t forget the cleanup function.
- What are keys in React and why are they important? Explain how keys help React identify which items changed in a list.
- What is lifting state up? Use a parent-child example. When shared data is needed by two components, the state gets transferred to their common parent.
- What is prop drilling and how can it be avoided? Explain how context can be used in place of passing props down many nested components.
- What are controlled and uncontrolled components? Controlled components use React state. Uncontrolled ones use refs and DOM directly.
- How is React different from other frameworks such as Angular or Vue? Describe its one-way data flow and component-based organization.
- What is a higher-order component (HOC)? It is a function that receives a component and returns a new one with additional capabilities.
- Why do we use
React.memo?
It prevents unwanted re-renders of functional components when props haven’t altered.
Attempt to write brief answers and describe them verbally. If you can teach it, you really know it!
5. Learn a Little Redux (Even if You Hate It)
We know—Redux is confusing initially. But it keeps appearing in quite a few React interview stages.
Begin by knowing why Redux is there. It makes state management global when passing props becomes untidy.
Understand about actions, reducers, and the store. You do not have to be extremely deep—but know how data flows.
Practice writing a basic counter with Redux. Then transition to a shopping cart application. It’s the ideal learning curve.
Current Redux employs Redux Toolkit. It makes many things easier. If you’re able, flaunt that knowledge—it impresses interviewers.
Prepare for questions such as: “When would you use Redux instead of useContext
?” Have a good answer ready.
Even with just the basics of Redux, you can have an added advantage in a tough React interview.
6. Understand How React Interacts with APIs and Routing
They tend to ask you in interviews to make small projects using APIs. So, knowing how to retrieve data is really crucial.
Call APIs using fetch or Axios. Also, handle the loading and error states—demonstrates real-world preparedness.
Understand CORS problems and how to resolve them. You don’t have to be a guru, just understand how to describe the error.
Routing also comes into play. Use React Router to change pages within your app. Study useParams, useNavigate, and route nesting.
Create a mini blog app with posts, comments, and a dedicated page for every post. That’s a treasure trove of interview practice.
7. Don’t Just Code—Explain Your Thinking Clearly
React interviews are not only about the correct output. They are concerned with how you approach problems and describe the solution.
Walk through your reasoning. “First, I’m going to fetch the data. Then I’m going to render it. Then I’m going to manage loading states.”
Whiteboard or code talk. Better yet, explain it to someone else.
Tell them why you used a specific hook or pattern. It demonstrates that you know React intimately—not simply on the surface.
Don’t freak out if you screw up. Interviews are less about perfection and more about problem-solving. Debugging is just part of the gig!
8. Bonus: Soft Skills Will Also Help You Nail the React Interview
Having the right tech skills is essential—but being confident, curious, and calm will make you stand out.
Smile, be friendly, and show enthusiasm for learning. This is React; it is ever-changing, so interviewers value flexible learners.
If something is unclear, ask away. It signals that you want to do things right rather than just taking everyone else’s word for it.
Be honest about what you do not know and discuss how you will find the answer. That will impress far more than attempting to bluff your way through.
Final Words: Practice, Patience, and Positivity
Secret to cracking a React interview? First, prepare, then keep calm, and finally, give yourself a little practice every day.
For a detailed guide on how to ace your React interview, check out this React Interview Guide 2025.
React means more than just code. It means making interactive and enjoyable experiences—and interviews are your gateway to this territory.
Keep learning, be curious, and don’t be afraid to make mistakes. Every great React dev began just where you stand right now.
Good luck—this is so yours!